Learn to Integrate Firebase Authentication With your Nuxt JS project
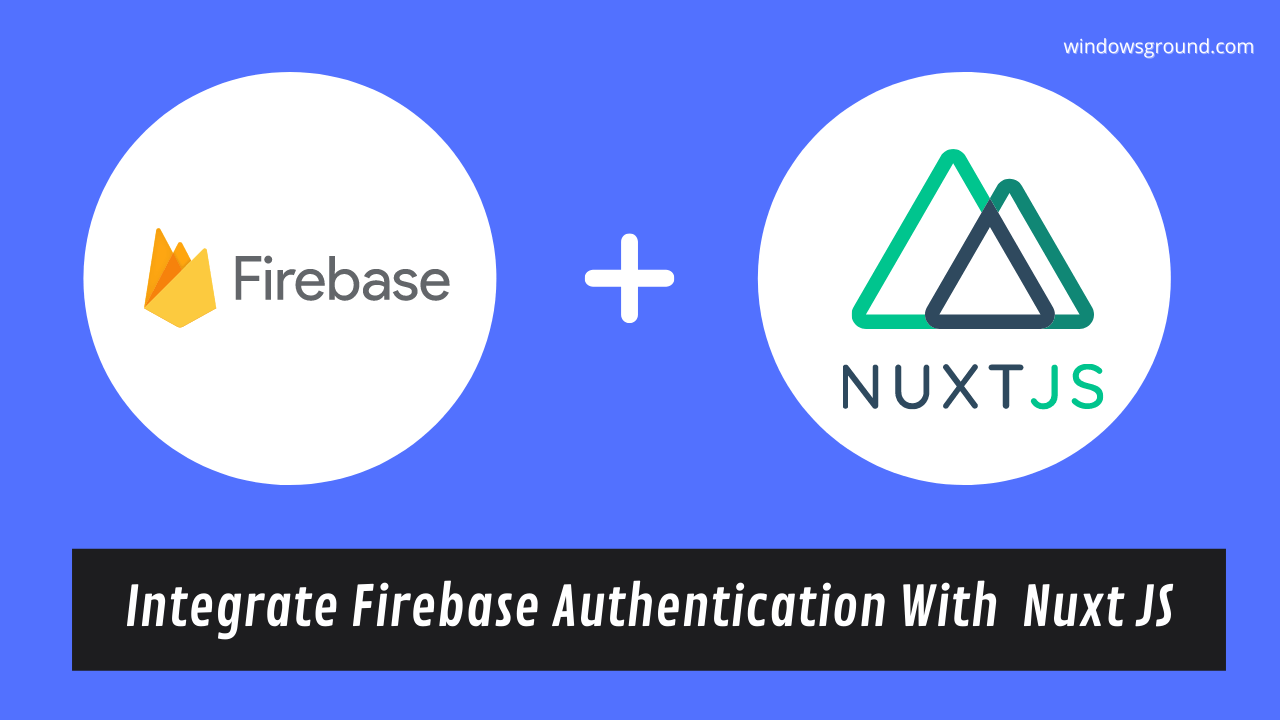
In this guide, you are going to learn about how to use Google’s firebase authentication service in your Nuxt Js app.
After this article, you will be able to create or Authenticate users with email and passwords in your app.
So if you want to or are thinking of trying to use firebase authentication in one of your Nuxt Js projects, You will love this article.
Requirements for authenticating with Firebase in Nuxt Js
- Node.js installed version (14.x or above) with yarn/npm package manager
- Code editor — I prefer Visual Studio Code
- Google account — we need this to use Firebase
- Basic knowledge of Nuxt Js— I won’t recommend this tutorial for complete beginners in Nuxt Js
If you face any issues throughout the tutorial, you can refer to the code in the GitHub repository.
Table of Contents
What is firebase Authentication?
Firebase is a platform developed by Google. Firebase is a Backend as a Service (BaaS) that provides a variety of services for web and mobile app development.
Such as:
- User authentication
- Scalable databases
- Cloud messaging
- Cloud code functions
- Social media Integration
- File storage
and so much more.
And Firebase Authentication is one of those services of firebase which allows you to implement authentication in your web app easily and thus improve security of your web app.
Using Firebase Authentication in a Nuxt Js project
Now let’s Start:
First of all, we’ll set up our Firebase project.
To do that go to firebase.google.com and login in with our google account.
Click on create a project, enter any name, accept the terms and click on continue.
After that untick Enable Google Analytics for this project (Because we don’t need google analytics for our project) and click on create project.
Wait for a few seconds for it to finish up and then click on continue
.
Now from the dashboard, click on this icon (marked below) to create an app for the web.
Now type any name for your web app and click on Register app
Now you will be presented with this screen, where you can see your API key and other personal information, make sure to keep it private.
Here you need to copy these 6 things and save it in a notepad or something because you are going to use them in the future.
apiKey: '<apiKey>',
authDomain: '<authDomain>',
projectId: '<projectId>',
storageBucket: '<storageBucket>',
messagingSenderId: '<messagingSenderId>',
appId: '<appId>',
measurementId: '<measurementId>'
After saving these details, click on continue to console.
Now we have created the app in firebase, we are going to use firebase authentication service with email and password.
Click on Build and then click on the Authentication tab.
Now click on get started
Here click on Email/password
Enable the top one and click one save
That’s it, now we are done with the firebase Part. Now let’s create a Nuxt js project.
Open your vscode editor and in the terminal type below command and hit enter. It will create a Nuxt Js project with name “nuxt-auth”
yarn create nuxt-app nuxt-auth
There will be a few configurations you need to select when creating the project, we will use some stuff along with Nuxt Js, so here are the configurations we need:
Note Press space to select Node js modules.
After that, we need to install a firebase in our project as well. To do that type the below command and hit enter.
yarn add firebase
And then install this Nuxt module as well by typing the below command and hitting enter.
yarn add @nuxtjs/firebase
After the successful installation, go to your Nuxt js project by typing cd nuxt-auth
command and press enter, and then type yarn dev
and hit enter to run the server.
This should fire up your browser and you should see the following screen:
Let’s clean up a little bit so that we can continue coding. In the Components folder, delete NuxtLogo.vue and Tutorial.vue. Once you delete these files, Remove the Tutorial component from index.vue, your index.vue file willlook like this:
<template>
</template>
<script>
export default {}
</script>
Now go to your nuxt.config.js file and
Replace this
modules: [
// https://go.nuxtjs.dev/axios
'@nuxtjs/axios'
],
To this:
modules: [
// https://go.nuxtjs.dev/axios
'@nuxtjs/axios',
[
'@nuxtjs/firebase',
{
config: {
apiKey: '<apiKey>',
authDomain: '<authDomain>',
projectId: '<projectId>',
storageBucket: '<storageBucket>',
messagingSenderId: '<messagingSenderId>',
appId: '<appId>',
measurementId: '<measurementId>'
},
services: {
auth: true // Just as example. Can be any other service.
}
}
]
],
Remember when I told you to copy those firebase API keys and other personal information? Now you need to paste that information here.
We have now connected Nuxt js to firebase. We can now use firebase authentication on our pages.
Creating a sign up page in Nuxt Js
First, we’ll create a sign-up feature that will let users sign up in our app with their email and password. And after that, we will redirect them to another page
Let’s start by making a simple sign-up HTML page in index.vue, and model the email and password input fields with an email and password variable so we can extract the data and then create a register button that will run a function called “createUser” when pressed.
The whole code will look like this:
In the scripts section, We declared two variables, email, and password which will model the data from those input fields we created above.
Then we will make a function named createUser which will call our auth instance from firebase which has a “createUserWithEmailAndPassword” method, this takes an email and password as arguments to create a new user object in firebase.
After this, we are just going to redirect the users to another page, name aftersignup
Now let’s create a page name aftersignup which we will show up after the registration of the user.
First, create a file name aftersignup.vue in your pages folder, And show whatever you want to show the user after the registration, for example :
The whole signup page will look like this:
And after the registration, it will look like this:
Now that we have created the signup page with the firebase authentication service, let’s make a login page.
Creating a Login page in Nuxt Js
First, we’ll create a login feature that will let users Sign in to our app with their email and password. And after the successful login, we will redirect them to another page
First, make a file name login.vue in the pages folder
then start making a simple login HTML page in login.vue, and model the email and password input fields with an email and password variable like we did in the registration page, and then create a login button that will run a function called “loginUser” when pressed.
The whole code will look like this:
Here we made a function named loginUser which will call our auth instance from firebase which has a “signInWithEmailAndPassword” method, this takes an email and password as arguments to Sign in.
After this, we are just going to redirect the users to another page, name afterlogin
Now let’s create a page name afterlogin which we will show up after the successful login of the user.
First create a file name afterlogin.vue in your pages folder, And then show whatever you want to show the user after the login, for example :
The whole login page will look like this:
And after the registration, it will look like this:
Lastly, run the below command to run the server to see all the changes.
yarn dev
And the app should be working fine.
Once you’re done with this build, You can try adding different authentication systems such as google authentication, Facebook authentication, etc, keep experimenting with the code.
If you have any errors or questions regarding the code, here’s the GitHub repository you can use for reference.
Also read:
Where are snipping tools screenshots saved Windows 10
[Fixed] minecraft stuck on white loading screen problem in windows 10 edition
Microsoft released Windows 10 Build 19044.1381 / 19043.1381 | Here is what is new
Quiz: Find out which Genshin impact character are you
Windows 10 bloatware list 2021 | Uninstall These Unnecessary Windows 10 programs
[Fixed] Antimalware Service Executable high memory in windows 10 (2021)